《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 3 Function Basics
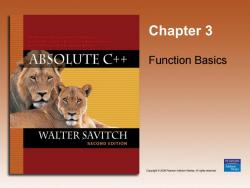
Chapter 3 ABSOLUTE C++ Function Basics WALTER SAVITCH SECOND EDITION PEARSON Copyright2006 Pearson Addison-Wesley All rights reserved
Chapter 3 Function Basics
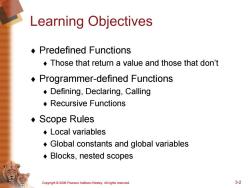
Learning Objectives Predefined Functions Those that return a value and those that don't Programmer-defined Functions Defining,Declaring,Calling ◆Recursive Functions ◆Scope Rules ◆Local variables Global constants and global variables Blocks,nested scopes Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-2
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-2 Learning Objectives ¨ Predefined Functions ¨ Those that return a value and those that don’t ¨ Programmer-defined Functions ¨ Defining, Declaring, Calling ¨ Recursive Functions ¨ Scope Rules ¨ Local variables ¨ Global constants and global variables ¨ Blocks, nested scopes
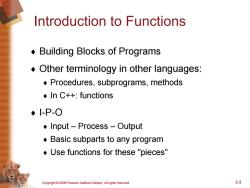
Introduction to Functions Building Blocks of Programs Other terminology in other languages: Procedures,subprograms,methods ◆lnC++:functions ◆-PO Input-Process-Output Basic subparts to any program Use functions for these "pieces" Copyright006 Pearson Addison-Wesley.All rights reserved. 3-3
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-3 Introduction to Functions ¨ Building Blocks of Programs ¨ Other terminology in other languages: ¨ Procedures, subprograms, methods ¨ In C++: functions ¨ I-P-O ¨ Input – Process – Output ¨ Basic subparts to any program ¨ Use functions for these "pieces
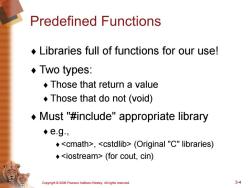
Predefined Functions Libraries full of functions for our use! ◆Two types: Those that return a value Those that do not (void) Must "#include"appropriate library ◆e.g, ,(Original "C"libraries) (for cout,cin) Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-4
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-4 Predefined Functions ¨ Libraries full of functions for our use! ¨ Two types: ¨Those that return a value ¨Those that do not (void) ¨ Must "#include" appropriate library ¨e.g., ¨ , (Original "C" libraries) ¨ (for cout, cin)

Using Predefined Functions Math functions very plentiful Found in library Most return a value (the "answer") Example:theRoot sqrt(9.0); ◆Components: sgrt name of library function theRoot variable used to assign "answer"to 9.0= argument or "starting input"for function ◆ln-P-O: ◆1=9.0 P="compute the square root" O=3,which is returned assigned to theRoot Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-5
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-5 Using Predefined Functions ¨ Math functions very plentiful ¨ Found in library ¨ Most return a value (the "answer") ¨ Example: theRoot = sqrt(9.0); ¨ Components: sqrt = name of library function theRoot = variable used to assign "answer" to 9.0 = argument or "starting input" for function ¨ In I-P-O: ¨ I = 9.0 ¨ P = "compute the square root" ¨ O = 3, which is returned & assigned to theRoot
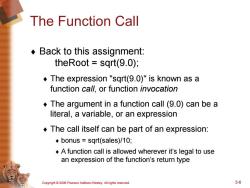
The Function Call Back to this assignment: theRoot sqrt(9.0); The expression "sqrt(9.0)"is known as a function call,or function invocation The argument in a function call(9.0)can be a literal,a variable,or an expression The call itself can be part of an expression: bonus sqrt(sales)/10; A function call is allowed wherever it's legal to use an expression of the function's return type Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-6
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-6 The Function Call ¨ Back to this assignment: theRoot = sqrt(9.0); ¨ The expression "sqrt(9.0)" is known as a function call, or function invocation ¨ The argument in a function call (9.0) can be a literal, a variable, or an expression ¨ The call itself can be part of an expression: ¨ bonus = sqrt(sales)/10; ¨ A function call is allowed wherever it’s legal to use an expression of the function’s return type
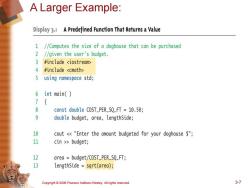
A Larger Example: Display 3.A Predefined Function That Returns a Value 1 //Computes the size of a doghouse that can be purchased 2 //given the user's budget. 3 #include 5 using namespace std; 6 int main() 7 8 const double COST_PER_SQ_FT 10.50; 9 double budget,area,lengthSide; 10 cout budget; 12 area budget/COST_PER_SQ_FT; 13 lengthSide sqrt(area); Copyright006 Pearson Addison-Wesley.All rights reserved. 3-7
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-7 A Larger Example:
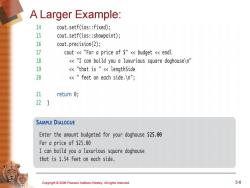
A Larger Example: 14 cout.setf(ios:fixed); 15 cout.setf(ios:showpoint); 16 cout.precision(2); 17 cout <<"For a price of $"<budget <endl 18 <<"I can build you a luxurious square doghouse\n" 19 <<"that is "<<lengthSide 20 <<"feet on each side.\n"; 21 return 0; 22} SAMPLE DIALOGUE Enter the amount budgeted for your doghouse $25.00 For a price of $25.00 I can build you a luxurious square doghouse that is 1.54 feet on each side. Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-8
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-8 A Larger Example:

More Predefined Functions ◆include Library contains functions like: ◆abs0 /Returns absolute value of an int ◆labs0 /Returns absolute value of a long int ·*fabs0∥Returns absolute value of a float *fabs()is actually in library ! ◆Can be confusing Remember:libraries were added after C++was "born,"in incremental phases Refer to appendices/manuals for details Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-9
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-9 More Predefined Functions ¨ #include ¨ Library contains functions like: ¨ abs() // Returns absolute value of an int ¨ labs() // Returns absolute value of a long int ¨ *fabs() // Returns absolute value of a float ¨ *fabs() is actually in library ! ¨ Can be confusing ¨ Remember: libraries were added after C++ was "born, " in incremental phases ¨ Refer to appendices/manuals for details
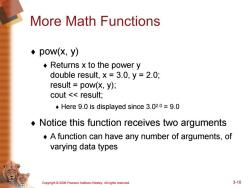
More Math Functions ◆poW(X,y) Returns x to the power y double result,x 3.0,y 2.0; result pow(x,y); cout <result; Here 9.0 is displayed since 3.02.0=9.0 Notice this function receives two arguments A function can have any number of arguments,of varying data types Copyright 2006 Pearson Addison-Wesley.All rights reserved. 3-10
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3-10 More Math Functions ¨ pow(x, y) ¨ Returns x to the power y double result, x = 3.0, y = 2.0; result = pow(x, y); cout << result; ¨ Here 9.0 is displayed since 3.02.0 = 9.0 ¨ Notice this function receives two arguments ¨ A function can have any number of arguments, of varying data types
按次数下载不扣除下载券;
注册用户24小时内重复下载只扣除一次;
顺序:VIP每日次数-->可用次数-->下载券;
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 1 C++ Basics.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 4 Parameters and Overloading.ppt
- 西安邮电大学:《信息论与编码》课程教学课件(PPT讲稿)第三章.ppt
- 西安邮电大学:《信息论与编码》课程教学课件(PPT讲稿)第二章 信源与信息熵.ppt
- 西安邮电大学:《信息论与编码》课程教学课件(PPT讲稿)第一章 绪论(主讲:王军选).ppt
- 《信息论与编码》课程教学资源(作业习题)第三章 信道与信道容量(含解答).pdf
- 《信息论与编码》课程教学资源(作业习题)自测题无答案.pdf
- 《信息论与编码》课程教学实验指导书.pdf
- 《信息论与编码》课程教学大纲 Element of Information Theory and Coding B.pdf
- 《信息论与编码》课程教学大纲 Element of Information Theory and Coding A.pdf
- 《信息安全概论》课程教学资源(PPT课件)第9章 信息安全标准与法律法规.ppt
- 《信息安全概论》课程教学资源(PPT课件)第2章 信息保密技术.ppt
- 《信息安全概论》课程教学资源(PPT课件)第7章 网络安全技术.ppt
- 《信息安全概论》课程教学资源(PPT课件)第8章 信息安全管理.ppt
- 《信息安全概论》课程教学资源(PPT课件)第6章 访问控制技术.ppt
- 《信息安全概论》课程教学资源(PPT课件)第5章 操作系统与数据库安全.ppt
- 《信息安全概论》课程教学资源(PPT课件)第1章 绪论.ppt
- 《信息安全概论》课程教学资源(PPT课件)第3章 信息认证技术.ppt
- 《信息安全概论》课程教学资源(PPT课件)第4章 信息隐藏技术.ppt
- 西安邮电大学:《信息安全概论》课程教学资源(教材书籍,共九章,人民邮电出版社,主编:张雪峰).pdf
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 2 Flow of Control.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 6 Structures and Classes.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 7 Constructors and Other Tools.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 8 Operator Overloading, Friends, and References.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 5 Arrays.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 11 Separate Compilation and Namespaces.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 12 Streams and File IO.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 10 Pointers and Dynamic Arrays.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 9 Strings.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 13 Inheritance.ppt
- 《C++面向对象程序设计》课程教学资源(PPT课件)Chapter 14 Polymorphism and Virtual Functions.ppt
- 《微机技术及应用》课程教学大纲 Microcmputer Technology and aplications.doc
- 《微型计算机技术及应用》课程电子教案(PPT教学课件,共十五章,完整版).pptx
- 《计算机导论》课程教学大纲 Computer Concepts.pdf
- 《计算机导论》课程教学课件(英文讲稿)1-a-Computer History+ Di Devices.pdf
- 《计算机导论》课程教学课件(英文讲稿)1-b-Digital Data Representation.pdf
- 《计算机导论》课程教学课件(英文讲稿)2-a-Computer Hardware.pdf
- 《计算机导论》课程教学课件(英文讲稿)2-b-Computer Hardware.pdf
- 《计算机导论》课程教学课件(英文讲稿)3-a-b-Computer Software.pdf
- 《计算机导论》课程教学课件(英文讲稿)4- operating system.pdf